If you’ve worked with APIs for any length of time, you’ve probably encountered the limitations of REST. And if you’re tired of dealing with multiple API endpoints just to fetch the data you need, GraphQL might just be the game-changer you’re looking for in web development.
Originally developed by Facebook, GraphQL is a query language for your APIs that lets you get exactly the data you need—nothing more, nothing less. But when is it useful, and when should you stick to good ol' REST? Let’s dive deep and demystify GraphQL in the context of software development and product development.
What Is GraphQL?
In simple terms, GraphQL is a query language for APIs and a runtime to execute those queries. It gives clients the ability to request precisely the data they need from the server in a single query.
In traditional REST APIs, you often have different endpoints for different resources. Let’s say you’re building an e-commerce app and you need to fetch user info, products, and orders. With REST, you’d hit three separate endpoints:
/users/123
/products/
/orders/
And often, the data returned contains fields you don’t need, inflating the payload. This is where GraphQL shines. With GraphQL, you can bundle all those requests into one query, specifying exactly which fields you want.
For example, here’s a GraphQL query to get user, product, and order information in one go:
With this query, the client gets exactly the requested data. No over-fetching, no under-fetching, just the essentials.

Flexible Queries: GraphQL allows clients to specify exactly what they need in a single request. No more multiple network requests or loading extra, unnecessary data.
Single Endpoint: Unlike REST, where each resource has its own endpoint, GraphQL operates through one endpoint—usually
/graphql
. This drastically simplifies API design.Versionless API: Since clients can ask for only the data they need, versioning becomes unnecessary. You can keep expanding your GraphQL schema without breaking old queries. REST often requires versioning (like
/v1/users
or/v2/products
) to handle new changes.Strongly-Typed Schema: GraphQL uses a strongly-typed schema to define the types of data that can be queried. This is great for both the server and client, as it allows self-documenting APIs and powerful validation.
Building a GraphQL API: Frameworks and Tools
So, how do you build a GraphQL API on the backend? The good news is that GraphQL can be implemented using many popular backend frameworks across different programming languages. Let's break down the most commonly used frameworks and tools:
Node.js (Express/Apollo Server):
Apollo Server is the most widely used library for building GraphQL APIs in Node.js. It integrates easily with Express, Koa, or other Node.js frameworks.
GraphQL.js is the core GraphQL library used to define types, queries, and mutations in JavaScript.
If you're already using Express for REST, migrating to GraphQL is straightforward. Just define your GraphQL schema and use the Apollo middleware to create a GraphQL endpoint.
Django (Graphene):
Graphene is a powerful Python library for building GraphQL APIs. It integrates seamlessly with Django, one of the most popular web frameworks in Python.
With Graphene-Django, you can quickly map your Django models to GraphQL types and define queries and mutations.
This makes Django + GraphQL an excellent choice for building data-heavy applications where relationships between models need to be queried efficiently.
Spring Boot (GraphQL Java):
GraphQL Java is the library you’ll use for building GraphQL APIs in Spring Boot (Java). It's widely used and supports the full GraphQL specification.
Spring Boot provides robust support for building enterprise-level applications, and adding GraphQL to the mix allows for dynamic and customizable queries that suit a wide range of use cases.
You can use Spring GraphQL to integrate easily with your existing Spring Data repositories.
Ruby on Rails (GraphQL-Ruby):
GraphQL-Ruby is a library that adds GraphQL functionality to Ruby on Rails applications.
It provides tools to define types, queries, and mutations, and integrates well with Rails models, making it easy to set up in a Rails app.
This is ideal if you're already using Rails and want to add GraphQL capabilities on top of your RESTful API.
Go (gqlgen):
In Go, the most popular library for GraphQL is gqlgen. It's a type-safe, code-generated approach to building GraphQL APIs.
Go is known for its performance and is well-suited for building scalable, high-performance APIs, and gqlgen helps ensure that your GraphQL API can handle a large number of queries efficiently.
When Should You Use GraphQL?
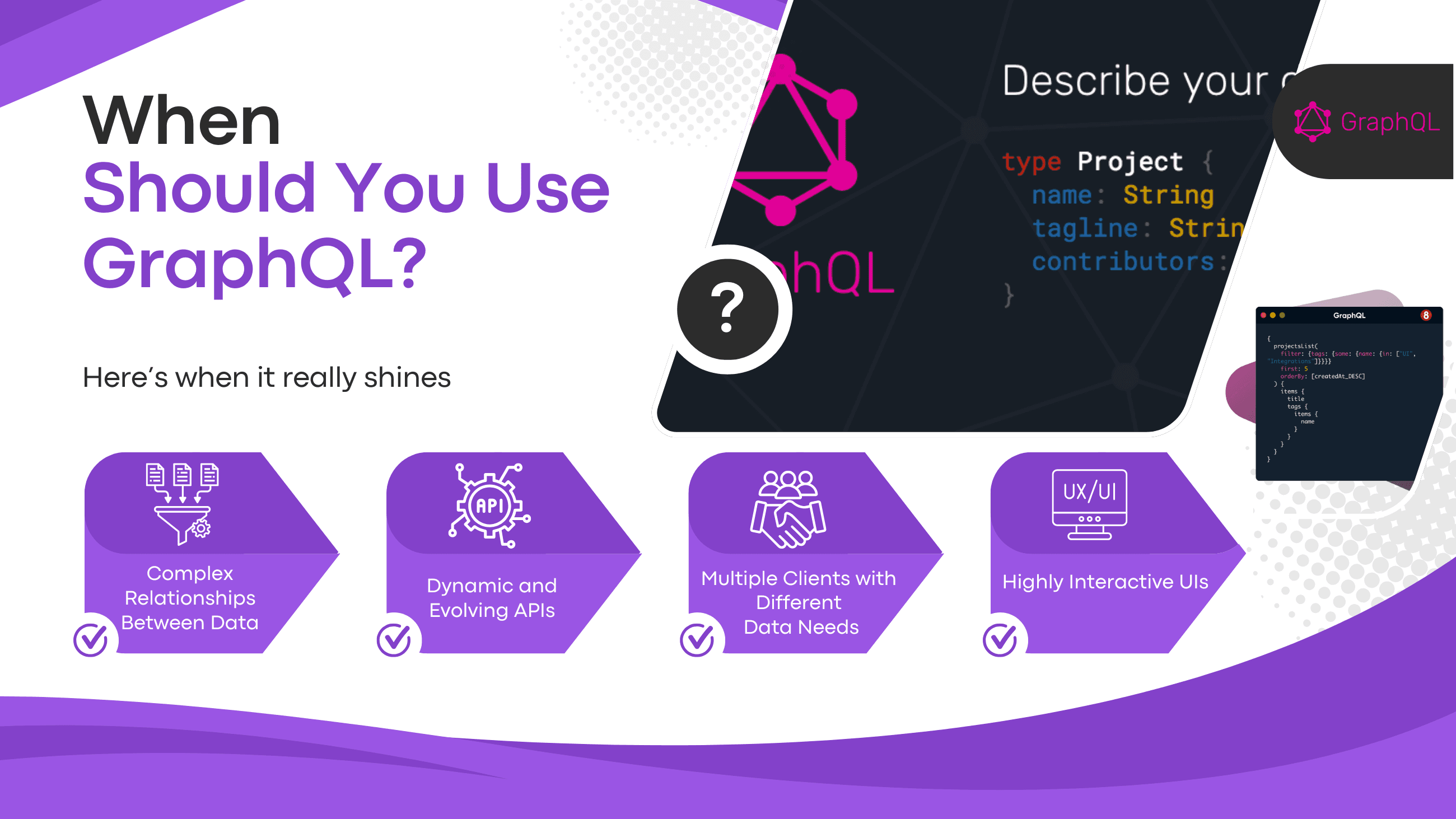
GraphQL isn’t a silver bullet. It’s fantastic for certain use cases but can be overkill for others. Here’s when it really shines:
Complex Relationships Between Data: If you have nested data or complex relationships (e.g., users and their orders, posts and their comments), GraphQL lets you retrieve everything in one query. This makes it ideal for apps with relational data.
Dynamic and Evolving APIs: If your API is constantly evolving—like a platform with rapidly changing requirements—GraphQL’s flexible querying and schema-based approach allow you to add fields without breaking existing clients.
Multiple Clients with Different Data Needs: When you have multiple consumers (like mobile and web clients) needing different slices of the same data, GraphQL lets each client request only what they need without impacting others.
Highly Interactive UIs: For applications like dashboards where the UI is data-intensive and users need to see real-time updates (e.g., analytics or social media feeds), GraphQL is perfect for managing large, complex queries efficiently.
When GraphQL Might Not Be the Best Choice
Simple APIs: If your API is simple and you’re serving static or minimal data, GraphQL might be overkill. REST still works great for basic CRUD operations where there’s little complexity.
Low-Traffic or Backend-Heavy Projects: If your app isn’t data-heavy and most of the logic happens on the backend, the overhead of setting up a GraphQL server might not be worth it.
Caching: REST integrates well with traditional HTTP caching mechanisms (like
GET
requests being cacheable). GraphQL’s flexibility makes caching more complex because every request can be different. You’d need to set up custom caching solutions.Learning Curve: GraphQL has a steeper learning curve than REST, especially if your team is already used to REST APIs. If you’re working on a tight schedule with a small team, sticking with REST might make more sense.
Who’s Using GraphQL?
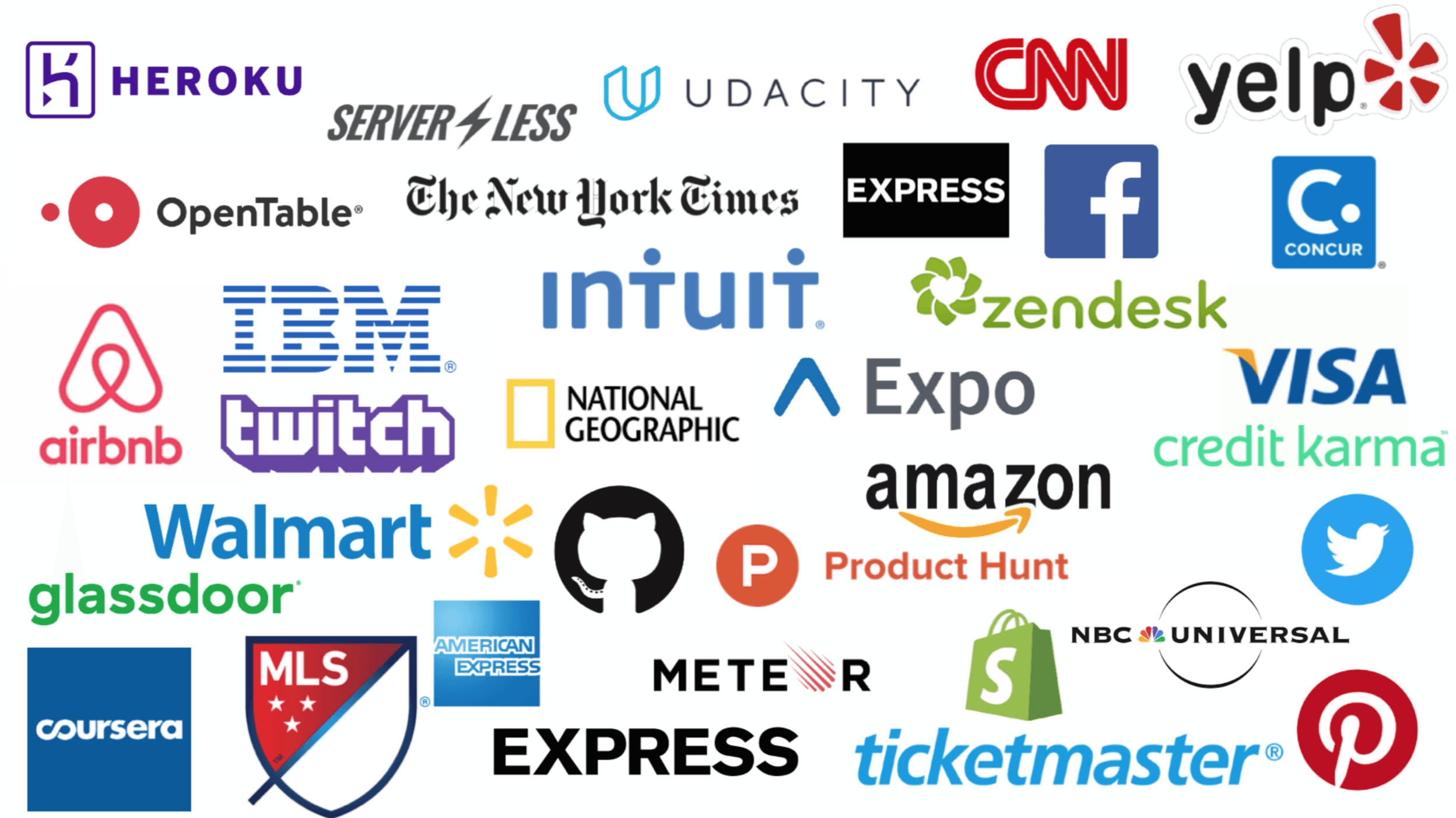
Some major tech companies and projects have embraced GraphQL due to its flexibility and power. Here are some examples:
Facebook: Unsurprisingly, since they developed it! Facebook’s mobile app fetches dynamic, multi-relational data using GraphQL.
GitHub: Their entire API is built on GraphQL, enabling developers to request data for users, repos, and commits all at once.
Shopify: Uses GraphQL to power its extensive API ecosystem, making it easier for developers to fetch product, order, and inventory data in a single request.
Twitter: Their backend APIs have been transitioning to GraphQL to handle complex data requests and deliver faster API responses.
Pinterest: Uses GraphQL to optimize its mobile app, enabling faster delivery of personalized content.
& Many more companies are using GraphQL

Discover More Insights
Continue learning with our selection of related topics. From AI to web development, find more articles that spark your curiosity.
AI
Jul 10, 2025
Agentic AI vs LLM vs Generative AI: Understanding the Key Differences
Confused by AI buzzwords? This guide breaks down the difference between AI, Machine Learning, Large Language Models, and Generative AI — and explains how they work together to shape the future of technology.
Tech
Jul 7, 2025
Next.js vs React.js - Choosing a Frontend Framework over Frontend Library for Your Web App
Confused between React and Next.js for your web app? This blog breaks down their key differences, pros and cons, and helps you decide which framework best suits your project’s goals
AI
Jun 28, 2025
Top AI Content Tools for SEO in 2025
This blog covers the top AI content tools for SEO in 2025 — including ChatGPT, Gemini, Jasper, and more. Learn how marketers and agencies use these tools to speed up content creation, improve rankings, and stay ahead in AI-powered search.
Performance Marketing
Apr 15, 2025
Top Performance Marketing Channels to Boost ROI in 2025
In 2025, getting leads isn’t just about running ads—it’s about building a smart, efficient system that takes care of everything from attracting potential customers to converting them.
Tech
Jun 16, 2025
Why Outsource Software Development to India in 2025?
Outsourcing software development to India in 2025 offers businesses a smart way to access top tech talent, reduce costs, and speed up development. Learn why TechTose is the right partner to help you build high-quality software with ease and efficiency.
Digital Marketing
Feb 14, 2025
Latest SEO trends for 2025
Discover the top SEO trends for 2025, including AI-driven search, voice search, video SEO, and more. Learn expert strategies for SEO in 2025 to boost rankings, drive organic traffic, and stay ahead in digital marketing.
AI & Tech
Jan 30, 2025
DeepSeek AI vs. ChatGPT: How DeepSeek Disrupts the Biggest AI Companies
DeepSeek AI’s cost-effective R1 model is challenging OpenAI and Google. This blog compares DeepSeek-R1 and ChatGPT-4o, highlighting their features, pricing, and market impact.
Web Development
Jan 24, 2025
Future of Mobile Applications | Progressive Web Apps (PWAs)
Explore the future of Mobile and Web development. Learn how PWAs combine the speed of native apps with the reach of the web, delivering seamless, high-performance user experiences
DevOps and Infrastructure
Dec 27, 2024
The Power of Serverless Computing
Serverless computing eliminates the need to manage infrastructure by dynamically allocating resources, enabling developers to focus on building applications. It offers scalability, cost-efficiency, and faster time-to-market.
Authentication and Authorization
Dec 11, 2024
Understanding OAuth: Simplifying Secure Authorization
OAuth (Open Authorization) is a protocol that allows secure, third-party access to user data without sharing login credentials. It uses access tokens to grant limited, time-bound permissions to applications.
Web Development
Nov 25, 2024
Clean Code Practices for Frontend Development
This blog explores essential clean code practices for frontend development, focusing on readability, maintainability, and performance. Learn how to write efficient, scalable code for modern web applications
Cloud Computing
Oct 28, 2024
Multitenant Architecture for SaaS Applications: A Comprehensive Guide
Multitenant architecture in SaaS enables multiple users to share one application instance, with isolated data, offering scalability and reduced infrastructure costs.
Technology
Sep 27, 2024
CSR vs. SSR vs. SSG: Choosing the Right Rendering Strategy for Your Website
CSR offers fast interactions but slower initial loads, SSR provides better SEO and quick first loads with higher server load, while SSG ensures fast loads and great SEO but is less dynamic.
Technology & AI
Sep 18, 2024
Introducing OpenAI O1: A New Era in AI Reasoning
OpenAI O1 is a revolutionary AI model series that enhances reasoning and problem-solving capabilities. This innovation transforms complex task management across various fields, including science and coding.
Tech & Trends
Sep 12, 2024
The Impact of UI/UX Design on Mobile App Retention Rates | TechTose
Mobile app success depends on user retention, not just downloads. At TechTose, we highlight how smart UI/UX design boosts engagement and retention.
Framework
Jul 21, 2024
Server Actions in Next.js 14: A Comprehensive Guide
Server Actions in Next.js 14 streamline server-side logic by allowing it to be executed directly within React components, reducing the need for separate API routes and simplifying data handling.